3. Configuration of gsplot#
Configuration provides a way to customize default keyword arguments of the functions defined in gsplot
. This is very useful when you need to plot multiple plots with the same parameters.
The configuration file can be described in json format. Each keyword needs to be referred from functions defined in gsplot
, and parameters can be passed to the function. At default, gsplot
will search for the configuration in the following directories:
Current working directory:
./gsplot.json
Userβs configuration directory:
~/.cofig/gsplot/gsplot.json
Userβs home directory:
~/gsplot.json
You can also specify the configuration file by using the gsplot.config_load function.
Note
Function that can be configured have a note about passed parameters in APIs.
Priority of Parameters#
The priority of the parameters is as follows:
Parameters passed to the function
Parameters passed to the configuration
Default parameters
%%{init: { "theme": "base", "themeVariables": { "primaryColor": "#ffffff", "primaryBorderColor": "#61AFC2", "edgeLabelBackground": "#ffffff", "tertiaryColor": "#61AFC2" }, "themeCSS": "marker path { fill: #61AFC2 !important; }" }}%% graph TD linkStyle default stroke:#61AFC2,stroke-width:1; Start([Start]) --> A[Parameters passed to the function?] A -->|Yes| F[Final Parameters] A -->|No| B[Parameters passed to the configuration?] B -->|Yes| F B -->|No| C[Default parameters] C --> F
Example of Axes with Configuration#
As a example, we will create a configuration file that sets parameters of the gsplot.axes. Arguments of gsplot.axes are following:
gsplot.axes(store = False, size = [5, 5], unit = "in", mosaic = "A", clear = True, ion = False)
If you set the configuration file like this:
{
"axes" : {
"size" : [10, 10],
"mosaic" : "AB"
}
}
And place this configuration file in the proper directory, gsplot
will read the configuration. If you create a plot with the following code:
gsplot.axes(store= True, mosaic = "ABC")
, this code is equivalent to the following code:
gsplot.axes(store = True, size = [10, 10], unit = "in", mosaic = "ABC", clear = True, ion = False)
Example#
Main Functions#
Function |
A Brief Overview |
---|---|
Load configuration file with a specific path |
|
Get dictionary of the configuration |
|
Get dictionary of configuration with a specific key |
Code#
from rich import print
import gsplot as gs
# gsplot provides a configuration file to set the default values of the plot
# gsplot will search for the configuration file in the following locations:
# 1. Current directory: ./gsplot.json
# 2. User config directory: ~/.config/gsplot/gsplot.json
# 3. Home directory: ~/gsplot.json
#
# You can also specify the configuration file path with the `config_load` function
config = gs.config_load(r"./gsplot.json")
# Check the configuration
config_dict = gs.config_dict()
print("\nconfig_dict:", config_dict)
axes_config = gs.config_entry_option("axes")
print("\naxes_config:", axes_config)
# Create a figure with the configuration
# Priority of the configuration
# 1. Direct arguments to the function
# 2. Configuration file
# 3. Default values
axs = gs.axes(store=True, size=(5, 5), mosaic="A")
gs.show("config")
Configuration#
{
"rich": {
"traceback": {}
},
"rcParams": {
"xtick.major.pad": 6,
"ytick.major.pad": 6
},
"axes": {
"ion": false,
"size": [
15.0,
5.0
],
"unit": "in",
"clear": false,
"mosaic": "ABC"
},
"show": {
"show": true
}
}
Plot#
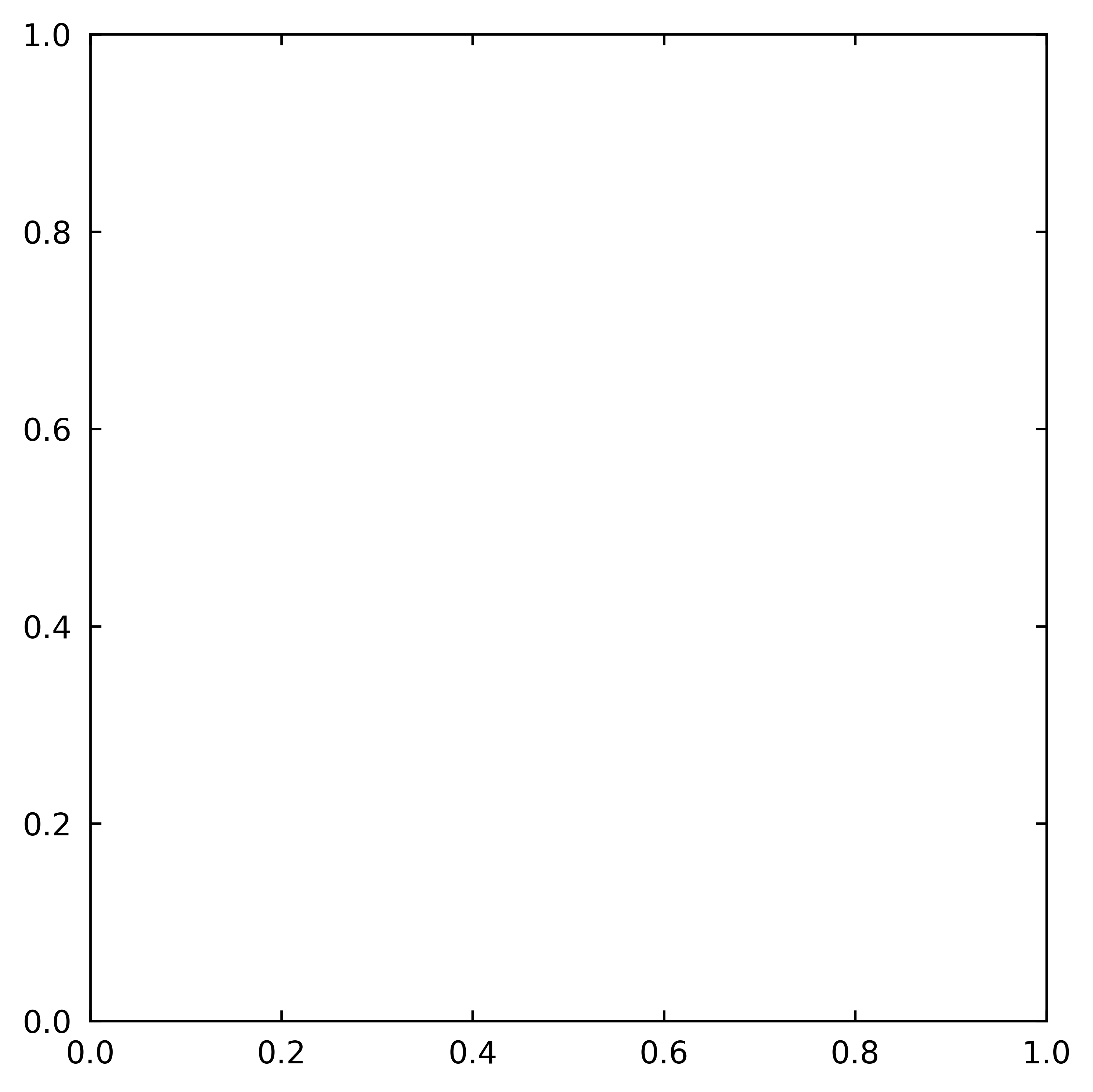
Output#
config_dict:
{
'rich': {'traceback': {}},
'rcParams': {'xtick.major.pad': 6, 'ytick.major.pad': 6},
'axes': {'ion': True, 'size': [15.0, 5.0], 'unit': 'in', 'clear': True, 'mosaic': 'ABC'},
'show': {'show': True}
}
axes_config:
{'ion': True, 'size': [15.0, 5.0], 'unit': 'in', 'clear': True, 'mosaic': 'ABC'}
config_dict:
{
'rich': {'traceback': {}},
'rcParams': {'xtick.major.pad': 6, 'ytick.major.pad': 6},
'axes': {'ion': False, 'size': [15.0, 5.0], 'unit': 'in', 'clear': False, 'mosaic': 'ABC'},
'show': {'show': True}
}
axes_config:
{'ion': False, 'size': [15.0, 5.0], 'unit': 'in', 'clear': False, 'mosaic': 'ABC'}